Axios vs. Fetch (2025 update): Which should you use for HTTP requests?
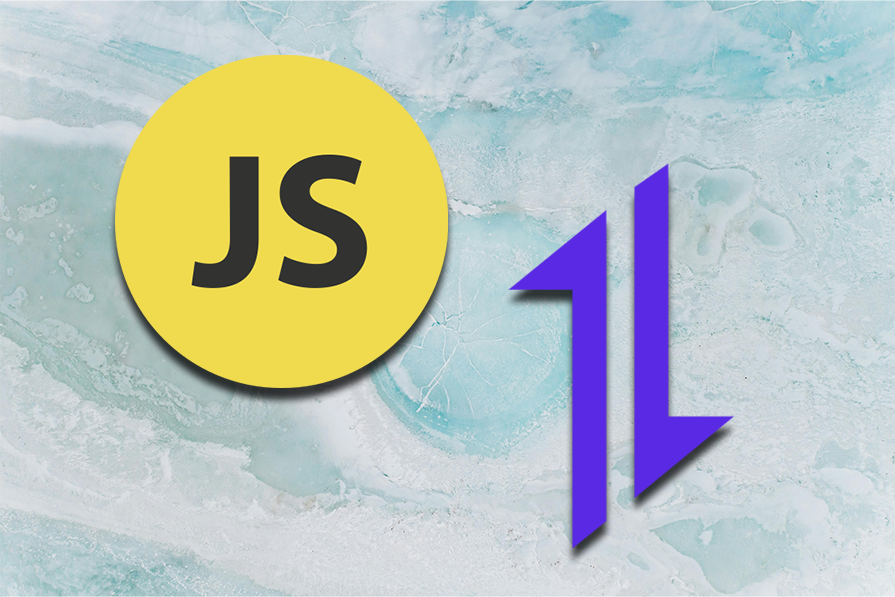
Table of Contents
- Introduction
- Comparison of Fetch API and Axios
- Using Axios for HTTP Requests
- Backward Compatibility
- HTTP Interceptors
- Error Handling
- Advanced Use Cases
- Handling Response Timeouts
- Cancelling Requests
- Streaming
- Best Practices for Different Use Cases
Introduction
When it comes to making HTTP requests, developers often debate between using the Fetch API and the Axios library. Both have their own strengths and weaknesses, and it's important to understand the differences between the two before deciding which one to use in your project.
Comparison of Fetch API and Axios
-
Origin:
- Fetch API: Native JavaScript API
- Axios: Third-party library
-
Installation:
- Fetch API: Natively available to browsers and Node.js v18+
- Axios: Requires npm install
-
JSON Parsing:
- Fetch API: Manual (need to use .json())
- Axios: Automatic
-
Error Handling:
- Fetch API: Minimal (only network errors)
- Axios: Comprehensive
-
Request Interceptors:
- Fetch API: Not available
- Axios: Available
-
Request Cancellation:
- Fetch API: Requires AbortController
- Axios: Built-in method
-
Platform Support:
- Fetch API: Once browser-only but now available in Node.js v18+
- Axios: Browser and Node.js
Using Axios for HTTP Requests
- To send a request with custom headers using Axios:
axios({ method: 'post', url: 'https://api.example.com', headers: { 'Authorization': 'Bearer token' }, data: { key: 'value' } });
Backward Compatibility
- Axios provides wide browser support, but if backward compatibility is your only concern, you can use Fetch API with a polyfill instead of an HTTP library.
HTTP Interceptors
- Axios allows you to intercept HTTP requests, which can be useful for adding custom logic or handling authentication.
Error Handling
- Fetch API and Axios handle errors differently. Axios provides a more comprehensive way of handling errors compared to Fetch API.
Advanced Use Cases
-
Handling Response Timeouts:
- Axios provides built-in support for handling response timeouts, while with Fetch API, it's more complex to implement.
-
Cancelling Requests:
- Both Axios and Fetch API support cancelling requests, but the implementation differs between the two.
-
Streaming:
- Fetch API and Axios offer different approaches to streaming requests, with Axios providing more flexibility.
Best Practices for Different Use Cases
- Based on your project requirements, choose between Fetch API and Axios for simple browser requests, large-scale API calls, retry logic, file uploads/downloads, building reusable HTTP utilities, and handling authenticated requests and tokens.