Create complex animation curves in CSS with the `linear()` easing function
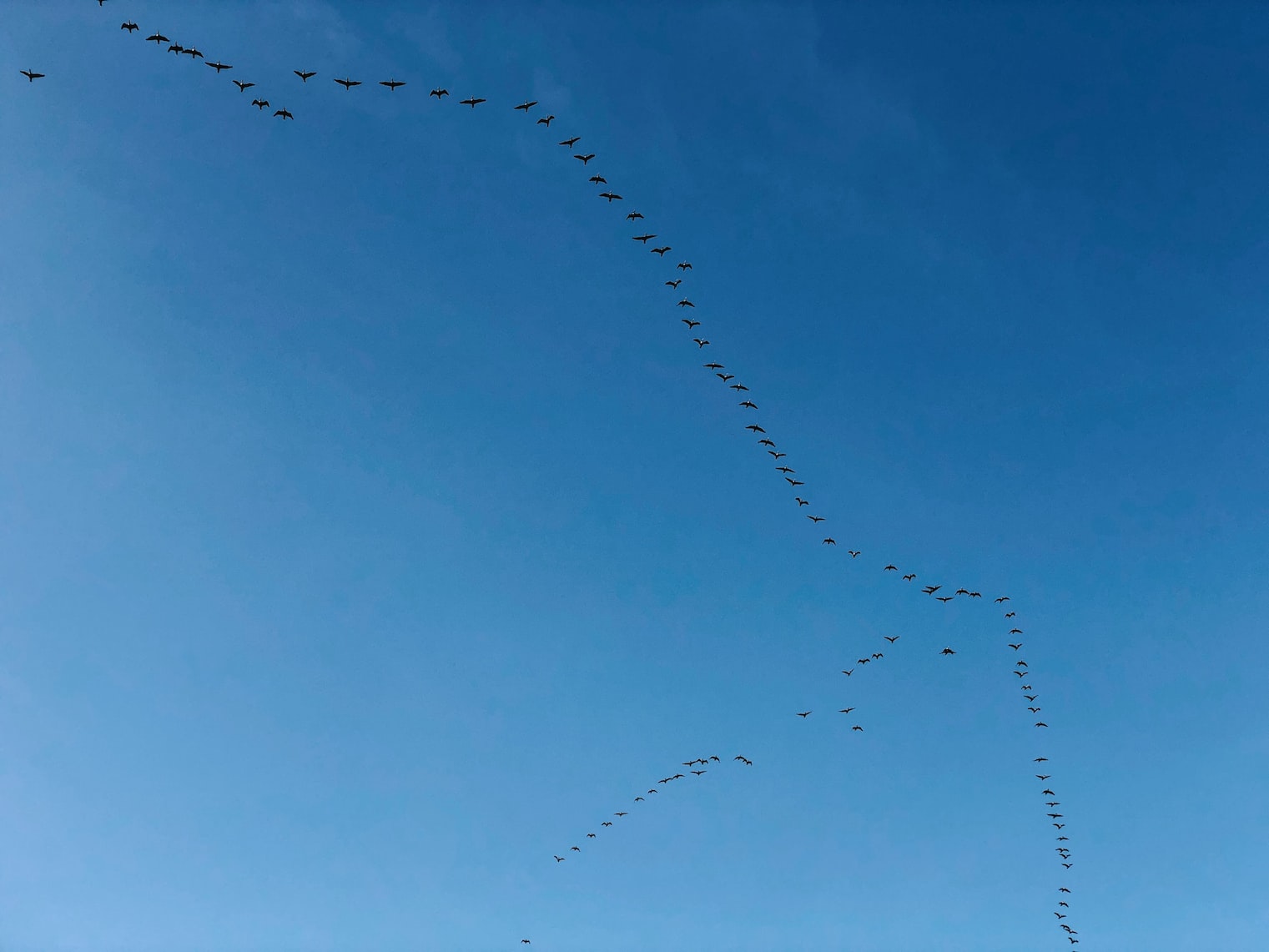
Table of Contents
- Easings in CSS
- An intro to linear()
- Creating complex curves with linear()
- A tool to help
- DevTools support
Easings in CSS
When animating or transitioning elements in CSS, you control the rate at which a value changes with an easing function using the transition-timing-function
and animation-timing-function
properties. There are several keywords available as presets in CSS, namely ease
, linear
, ease-in
, ease-out
, and ease-in-out
.
An intro to linear()
Creating complex curves such as bounce or spring effects is not possible in CSS, but thanks to linear()
you can now approximate them astonishingly well. This function accepts a number of stops, separated by commas. These stops are by default spread equidistantly.
Creating complex curves with linear()
While the examples above are very simple easings, you can use linear()
to recreate complex easing functions in a very simple manner, with the compromise of losing some precision. Take this bounce easing curve, a type of easing that cannot be expressed directly in CSS, defined using JavaScript:
function bounce(time) {
if (time < (1 / 2.75)) {
return 7.5625 * time * time;
} else if (time < (2 / 2.75)) {
return 7.5625 * (time -= (1.5 / 2.75)) * time + 0.75;
} else if (time < (2.5 / 2.75)) {
return 7.5625 * (time -= (2.25 / 2.75)) * time + 0.9375;
} else {
return 7.5625 * (time -= (2.625 / 2.75)) * time + 0.984375;
}
}
The curve can be simplified by adding a number of stops onto it. When passed into linear()
, the result is a curve that kinda looks like the original one, but is a bit rougher on the edges.
A tool to help
Manually creating the list of stops would be very cumbersome. The tool takes a JavaScript easing function or SVG curve as its input, and outputs the simplified curve using linear()
.
DevTools support
Available in DevTools is support to visualize and edit the result of linear()
. Click on the icon to show an interactive tooltip that lets you drag around the stops.