GitHub OAuth in your Python Flask app
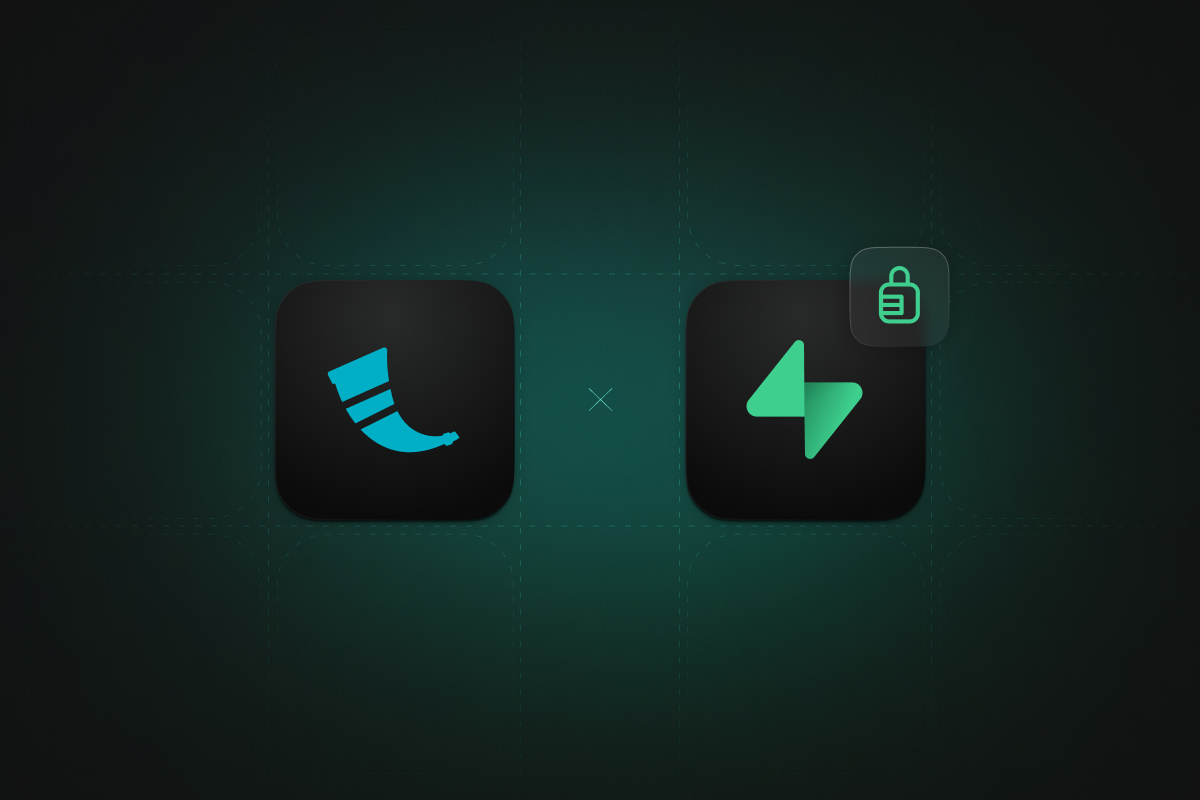
Supabase-py: GitHub OAuth Integration with Flask
Supabase-py is a Python library that allows you to integrate Supabase authentication into your Flask app using GitHub OAuth2.0. This tutorial will guide you through the process of setting up OAuth integration with GitHub in a Flask app using Supabase-py.
Prerequisites
Before getting started, make sure you have the following prerequisites:
- Familiarity with Flask app development
- A Supabase account
- Flask version 2.3.3 or later
Setup
- Install the Supabase-py library in your Flask app.
- Create a session storage file that extends the Supabase-py session storage class.
- Initialize the Supabase client in a separate file.
- Set up the sign-in with GitHub functionality.
- Create a callback route to handle the OAuth callback.
Session Storage
Create a file named session_storage.py
with the following content:
from supabase_py import create_client, Client, Storage
class FlaskSessionStorage(Storage):
def store_tokens(self, data):
session['supabase_tokens'] = data
def fetch_tokens(self):
return session.get('supabase_tokens')
def remove_tokens(self):
session.pop('supabase_tokens', None)
In this file, we extend the Storage
class from the Supabase-py library and override the methods to store, fetch, and remove session tokens. In this example, we are using Flask's session to store the tokens.
Client Initialization
Create a file named supabase_client.py
with the following content:
from flask import g
from supabase_py import create_client
def get_supabase_client():
if not hasattr(g, 'supabase_client'):
g.supabase_client = create_client(
'SUPABASE_URL',
'SUPABASE_KEY',
session_storage=FlaskSessionStorage,
)
return g.supabase_client
In this file, we define a function get_supabase_client()
that initializes the Supabase client. We check if the client is already stored in the global object g
, and if not, we create a new client with the Supabase URL, Supabase key, and our custom session storage class.
Sign-in with GitHub
To enable sign-in with GitHub, you can add the following route in your Flask app:
from flask import Flask, redirect, url_for
from supabase_client import get_supabase_client
app = Flask(__name__)
@app.route('/signin')
def signin():
supabase_client = get_supabase_client()
redirect_url = url_for('callback', _external=True)
auth_url = supabase_client.auth.sign_in_with_provider('github', redirect_url)
return redirect(auth_url)
In this route, we get the Supabase client using the get_supabase_client()
function. We specify the callback URL as a parameter in the sign_in_with_provider
method and redirect the user to the GitHub OAuth consent screen.
Callback Route
Create another route in your Flask app to handle the callback from GitHub:
@app.route('/callback')
def callback():
supabase_client = get_supabase_client()
code = request.args.get('code')
redirect_url = url_for('callback', _external=True)
supabase_client.auth.user().refresh_access_token()
tokens = supabase_client.auth.sign_in_with_authorization_code(code, redirect_url)
user = supabase_client.auth.user()
session['user_id'] = user['id']
return redirect(url_for('home'))
In this route, we retrieve the authorization code from the query parameters and use it to exchange for a session token. We refresh the access token, sign in with the authorization code, and store the user ID in the session. Finally, we redirect the user to the home page.
Conclusion
In this tutorial, we learned how to integrate GitHub OAuth2.0 into a Flask app using Supabase-py. We covered setting up session storage, initializing the Supabase client, enabling sign-in with GitHub, and handling the callback from GitHub.
For more information, check out the Supabase-py reference documentation and Supabase-py GitHub repository.