Refactor your code with C# collection expressions
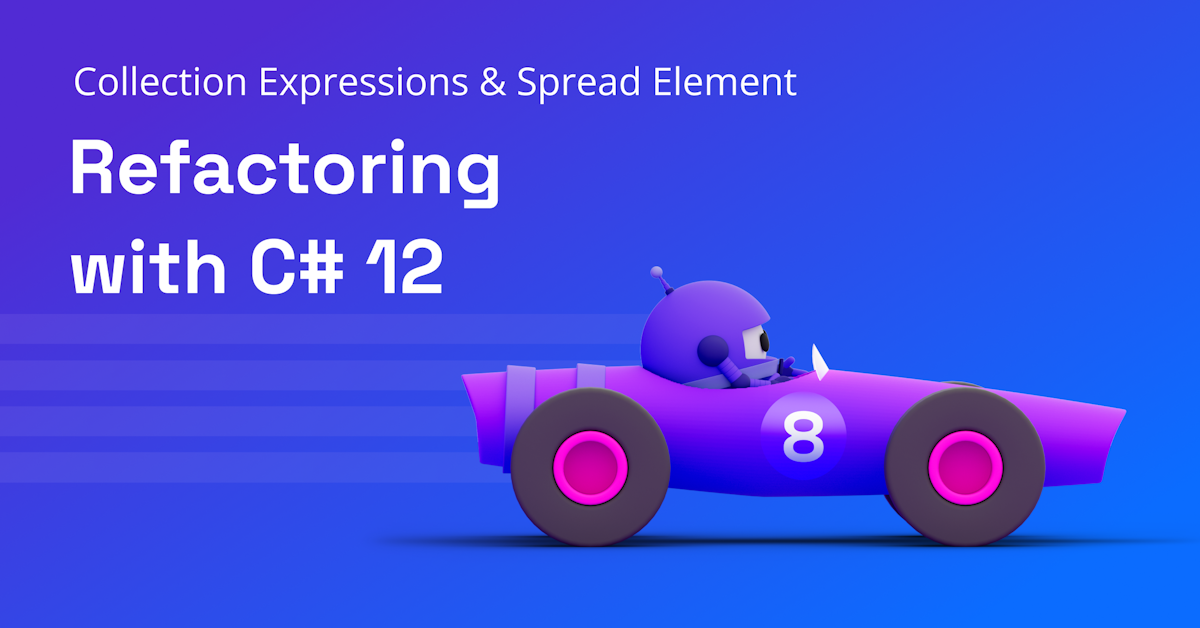
Refactor your code with C# collection expressions
Collection Initializers
- Different ways to initialize an array of integers are functionally equivalent with the compiler generating identical code.
- You must declare the type because a collection expression doesn't have a natural type.
Collection Expression Variations
- Empty collection expression:
new int[] { }
- Single element collection:
new int[] { 42 }
- Spread element to include elements of another collection:
new int[] { 1, 2, 3, 4, 5, 6 }
Supported Collection Types
- Collection expressions can be used with various target types.
Refactoring Scenarios
- Initializing empty collections that declare non-nullable collection types.
- Using spread element syntax for concatenating collections.
Summary
Collection expressions in C# provide a consistent and efficient way to initialize collections regardless of their target type. They support various collection types and can be used in different scenarios for refactoring code.