Using CORS in Next.js to handle cross-origin requests
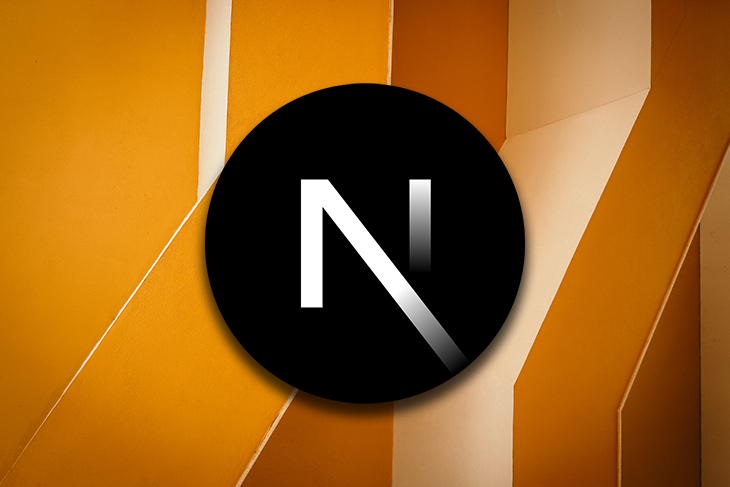
Summary
This article explores the concept of Cross-Origin Resource Sharing (CORS) in the context of Next.js. It explains why CORS is necessary for handling cross-origin requests and outlines the prerequisites for configuring CORS in a Next.js application. The article then provides step-by-step instructions on how to enable CORS for all API routes in Next.js by setting CORS headers using the config
key in next.config.js
. General tips and tricks for using CORS in Next.js are also provided, such as using environment variables for configuration and writing custom logic for permitting multiple CORS origins. The article concludes by emphasizing the importance of CORS in backend applications and how setting CORS headers in Next.js can ensure secure cross-origin communication.
Prerequisites for learning to configure CORS in Next.js
- Basic understanding of Next.js and API routes
- Familiarity with HTTP headers and cross-origin communication
Enabling CORS for all API routes in Next.js
- Create a file in the appropriate folder (
api
,pages/api
, orsrc/api
) callednext.config.js
(or use an existing one). - Inside the file, export a function that returns an array of objects representing the CORS policy.
- Configure the CORS policy according to your specific needs. Example configuration:
module.exports = {
// ...
async headers() {
return [
{
source: '/api/:path*',
headers: [
{
key: 'Access-Control-Allow-Origin',
value: '*',
},
],
},
];
},
};
- Save the file and run your Next.js application.
CORS headers in action
After configuring CORS in Next.js, the browser is now allowed to execute cross-origin requests. To see this in action, you can inspect the response headers in your browser's developer tools.
General tips for using CORS in Next.js
- Use environment variables for configuration: Instead of hardcoding CORS origins in the
next.config.js
file, you can use environment variables for more flexibility. - Handling multiple CORS origins: If you need to permit multiple but not all CORS origins, you will need to write custom logic in the CORS policy function.
Conclusion
In conclusion, CORS is an important security measure for backend applications, including those built with Next.js. By configuring CORS headers in Next.js, you can enable secure cross-origin communication and ensure that resources can be accessed from different domains. Understanding how to set up CORS in Next.js is crucial for building robust and secure applications.