Using react-arborist to create tree components for React
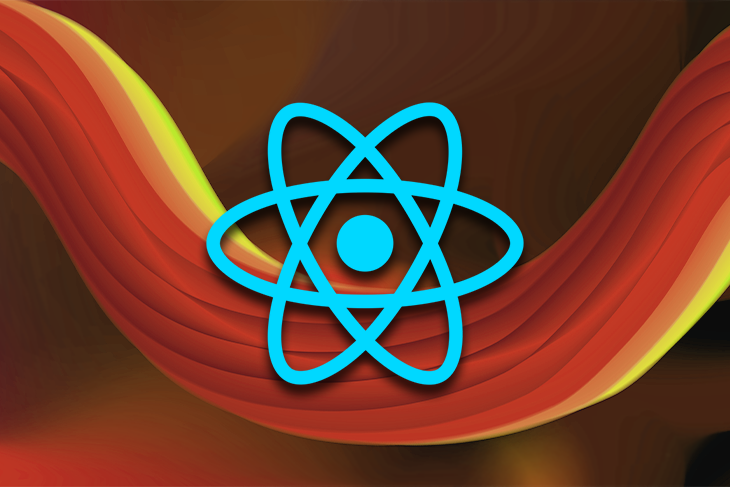
Creating a component
- To create a tree view component in a React project, we will use the react-arborist library.
- First, install the library using
npm install react-arborist
. - Additionally, install an icon library for React, such as
npm install react-icons
.
Using the library
- Import the necessary components from the react-arborist library.
- Use the
<Tree/>
component to render the tree view. - Pass the data for the tree nodes as an array of objects to the
nodes
prop. - Customize the appearance and behavior of the tree nodes using the available props and APIs.
Tree views and accessibility
- Tree views are UI components that present hierarchical data in a structured manner.
- They are commonly used for displaying file and folder structures, organizational hierarchies, and other nested data.
- When implementing tree views, it's important to consider accessibility guidelines, such as providing keyboard navigation and ARIA attributes.
Controlled and uncontrolled components
- The react-arborist library offers both controlled and uncontrolled components.
- In an uncontrolled component, the library handles the state internally and provides default behavior.
- In a controlled component, the state is controlled by the parent component, allowing for custom behavior and handling.
Setting up the component props
- Pass the
nodes
prop to the<Tree/>
component to define the data for the tree nodes. - Each node should be an object with properties such as
name
,icon
, andchildren
. - Use the
isOpen
prop to control whether a branch node is expanded or collapsed. - Customize the appearance of the nodes using the
renderer
prop.
Customizing the nodes in an uncontrolled component
- Use the
renderer
prop to create a custom node appearance. - Access the node data, state, and various APIs provided by the library within the custom renderer.
- Implement custom logic, such as toggling the node's open state or checking if it is a branch node.
Creating a controlled component
- To create a controlled component, manage the state of the tree nodes in the parent component.
- Pass the nodes data and related state to the
<Tree/>
component using props. - Handle events, such as node expansion or selection, in the parent component and update the state accordingly.
Summary
In this lesson, we learned how to use the react-arborist library to create tree view components in a React project. We explored the options for customizing the appearance and behavior of the tree nodes, as well as the differences between controlled and uncontrolled components. Additionally, we highlighted the importance of accessibility considerations when implementing tree views.